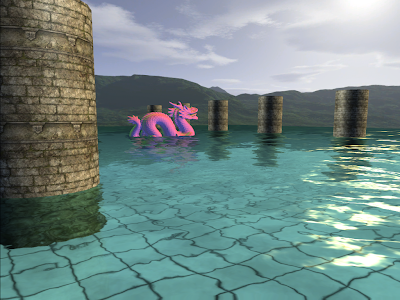
I had some requests awhile back for a more independent water effect that I had provided in my camera animation tutorials. And just the other day I remembered that I had totally forgot about this (doh!). So here is a DrawableGameComponent for the water effect. Have a look here to see it in action.
To setup the component we need to fill out a WaterOptions object that will be passed to the water component.
WaterOptions options = new WaterOptions();
options.Width = 257;
options.Height = 257;
options.CellSpacing = 0.5f;
options.WaveMapAsset0 = "Textures/wave0";
options.WaveMapAsset1 = "Textures/wave1";
options.WaveMapVelocity0 = new Vector2(0.01f, 0.03f);
options.WaveMapVelocity1 = new Vector2(-0.01f, 0.03f);
options.WaveMapScale = 2.5f;
options.WaterColor = new Vector4(0.5f, 0.79f, 0.75f, 1.0f);
options.SunColor = new Vector4(1.0f, 0.8f, 0.4f, 1.0f);
options.SunDirection = new Vector3(2.6f, -1.0f, -1.5f);
options.SunFactor = 1.5f;
options.SunPower = 250.0f;
mWaterMesh = new Water(this);
mWaterMesh.Options = options;
mWaterMesh.EffectAsset = "Shaders/Water";
mWaterMesh.World = Matrix.CreateTranslation(Vector3.UnitY * 2.0f);
mWaterMesh.RenderObjects = DrawObjects;
So here will fill out various options such as width and height, cell spacing, the normal map asset names, etc. We then create the water component, and assign it the options object. We then provide the filename of the Water.fx shader, the water's position and we then assign its RenderObjects delegate a function that will be used to draw the objects in your scene.
The component tries to be relatively independent of how your represent your game objects. All that it asks for is that you provide a function that takes a reflection matrix. This function should go through the objects that you want to be reflected/refracted and combine the reflection matrix with the object's world matrix.
Here's an example of what your DrawObjects() function might look like.
private void DrawObjects(Matrix reflMatrix)mWaterMesh.RenderObjects is the delegate that has the signature of: public delegate void RenderObjects(Matrix reflectionMatrix);
{
foreach (DrawableGameComponent mesh in Components)
{
Matrix oldWorld = mesh.World;
mesh.World = oldWorld * reflMatrix;
mesh.Draw(mGameTime);
mesh.World = oldWorld;
}
}
Basically this function should just go through your game objects and render them.
Lastly, before you draw your objects in the scene, you need to send to the water component the ViewProjection matrix and the camera's position by using WaterMesh.SetCamera(). And you need to call WaterMesh.UpdateWaterMaps() to update the reflection and refraction maps. After this, you can clear your framebuffer and draw your objects. For how this effect looks you can take a look at my camera animation tutorials.
Water GameComponent:
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Content;
namespace WaterSample
{
//delegate that the water component to call to render the objects in the scene
public delegate void RenderObjects(Matrix reflectionMatrix);
/// <summary>
/// Options that must be passed to the water component before Initialization
/// </summary>
public class WaterOptions
{
//width and height must be of the form 2^n + 1
public int Width = 257;
public int Height = 257;
public float CellSpacing = .5f;
public float WaveMapScale = 1.0f;
public int RenderTargetSize = 512;
//offsets for the texcoords of the wave maps updated every frame
public Vector2 WaveMapOffset0;
public Vector2 WaveMapOffset1;
//the direction to offset the texcoords of the wave maps
public Vector2 WaveMapVelocity0;
public Vector2 WaveMapVelocity1;
//asset names for the normal/wave maps
public string WaveMapAsset0;
public string WaveMapAsset1;
public Vector4 WaterColor;
public Vector4 SunColor;
public Vector3 SunDirection;
public float SunFactor;
public float SunPower;
}
/// <summary>
/// Drawable game component for water rendering. Renders the scene to reflection and refraction
/// maps that are projected onto the water plane and are distorted based on two scrolling normal
/// maps.
/// </summary>
public class Water : DrawableGameComponent
{
#region Fields
private RenderObjects mDrawFunc;
//vertex and index buffers for the water plane
private VertexBuffer mVertexBuffer;
private IndexBuffer mIndexBuffer;
private VertexDeclaration mDecl;
//water shader
private Effect mEffect;
private string mEffectAsset;
//camera properties
private Vector3 mViewPos;
private Matrix mViewProj;
private Matrix mWorld;
//maps to render the refraction/reflection to
private RenderTarget2D mRefractionMap;
private RenderTarget2D mReflectionMap;
//scrolling normal maps that we will use as a
//a normal for the water plane in the shader
private Texture mWaveMap0;
private Texture mWaveMap1;
//user specified options to configure the water object
private WaterOptions mOptions;
//tells the water object if it needs to update the refraction
//map itself or not. Since refraction just needs the scene drawn
//regularly, we can:
// --Draw the objects we want refracted
// --Resolve the back buffer and send it to the water
// --Skip computing the refraction map in the water object
private bool mGrabRefractionFromFB = false;
private int mNumVertices;
private int mNumTris;
#endregion
#region Properties
public RenderObjects RenderObjects
{
set { mDrawFunc = value; }
}
/// <summary>
/// Name of the asset for the Effect.
/// </summary>
public string EffectAsset
{
get { return mEffectAsset; }
set { mEffectAsset = value; }
}
/// <summary>
/// The render target that the refraction is rendered to.
/// </summary>
public RenderTarget2D RefractionMap
{
get { return mRefractionMap; }
set { mRefractionMap = value; }
}
/// <summary>
/// The render target that the reflection is rendered to.
/// </summary>
public RenderTarget2D ReflectionMap
{
get { return mReflectionMap; }
set { mReflectionMap = value; }
}
/// <summary>
/// Options to configure the water. Must be set before
/// the water is initialized. Should be set immediately
/// following the instantiation of the object.
/// </summary>
public WaterOptions Options
{
get { return mOptions; }
set { mOptions = value; }
}
/// <summary>
/// The world matrix of the water.
/// </summary>
public Matrix World
{
get { return mWorld; }
set { mWorld = value; }
}
#endregion
public Water(Game game) : base(game)
{
}
public override void Initialize()
{
base.Initialize();
//build the water mesh
mNumVertices = mOptions.Width * mOptions.Height;
mNumTris = (mOptions.Width - 1) * (mOptions.Height - 1) * 2;
VertexPositionTexture[] vertices = new VertexPositionTexture[mNumVertices];
Vector3[] verts;
int[] indices;
GenTriGrid(mOptions.Height, mOptions.Width, mOptions.CellSpacing, mOptions.CellSpacing,
Vector3.Zero, out verts, out indices);
//copy the verts into our PositionTextured array
for (int i = 0; i < mOptions.Width; ++i)
{
for (int j = 0; j < mOptions.Height; ++j)
{
int index = i * mOptions.Width + j;
vertices[index].Position = verts[index];
vertices[index].TextureCoordinate = new Vector2((float)j / mOptions.Width, (float)i / mOptions.Height);
}
}
mVertexBuffer = new VertexBuffer(Game.GraphicsDevice,
VertexPositionTexture.SizeInBytes * mOptions.Width * mOptions.Height,
BufferUsage.WriteOnly);
mVertexBuffer.SetData(vertices);
mIndexBuffer = new IndexBuffer(Game.GraphicsDevice, typeof(int), indices.Length, BufferUsage.WriteOnly);
mIndexBuffer.SetData(indices);
mDecl = new VertexDeclaration(Game.GraphicsDevice, VertexPositionTexture.VertexElements);
}
protected override void LoadContent()
{
base.LoadContent();
mWaveMap0 = Game.Content.Load<Texture2D>(mOptions.WaveMapAsset0);
mWaveMap1 = Game.Content.Load<Texture2D>(mOptions.WaveMapAsset1);
PresentationParameters pp = Game.GraphicsDevice.PresentationParameters;
SurfaceFormat format = pp.BackBufferFormat;
MultiSampleType msType = pp.MultiSampleType;
int msQuality = pp.MultiSampleQuality;
mRefractionMap = new RenderTarget2D(Game.GraphicsDevice, mOptions.RenderTargetSize, mOptions.RenderTargetSize,
1, format, msType, msQuality);
mReflectionMap = new RenderTarget2D(Game.GraphicsDevice, mOptions.RenderTargetSize, mOptions.RenderTargetSize,
1, format, msType, msQuality);
mEffect = Game.Content.Load<Effect>(mEffectAsset);
//set the parameters that shouldn't change.
//Some of these might need to change every once in awhile,
//move them to updateEffectParams if you need that functionality.
if (mEffect != null)
{
mEffect.Parameters["WaveMap0"].SetValue(mWaveMap0);
mEffect.Parameters["WaveMap1"].SetValue(mWaveMap1);
mEffect.Parameters["TexScale"].SetValue(mOptions.WaveMapScale);
mEffect.Parameters["WaterColor"].SetValue(mOptions.WaterColor);
mEffect.Parameters["SunColor"].SetValue(mOptions.SunColor);
mEffect.Parameters["SunDirection"].SetValue(mOptions.SunDirection);
mEffect.Parameters["SunFactor"].SetValue(mOptions.SunFactor);
mEffect.Parameters["SunPower"].SetValue(mOptions.SunPower);
mEffect.Parameters["World"].SetValue(mWorld);
}
}
public override void Update(GameTime gameTime)
{
float timeDelta = (float)gameTime.ElapsedGameTime.TotalSeconds;
mOptions.WaveMapOffset0 += mOptions.WaveMapVelocity0 * timeDelta;
mOptions.WaveMapOffset1 += mOptions.WaveMapVelocity1 * timeDelta;
if (mOptions.WaveMapOffset0.X >= 1.0f mOptions.WaveMapOffset0.X <= -1.0f)
mOptions.WaveMapOffset0.X = 0.0f;
if (mOptions.WaveMapOffset1.X >= 1.0f mOptions.WaveMapOffset1.X <= -1.0f)
mOptions.WaveMapOffset1.X = 0.0f;
if (mOptions.WaveMapOffset0.Y >= 1.0f mOptions.WaveMapOffset0.Y <= -1.0f)
mOptions.WaveMapOffset0.Y = 0.0f;
if (mOptions.WaveMapOffset1.Y >= 1.0f mOptions.WaveMapOffset1.Y <= -1.0f)
mOptions.WaveMapOffset1.Y = 0.0f;
}
public override void Draw(GameTime gameTime)
{
UpdateEffectParams();
Game.GraphicsDevice.Indices = mIndexBuffer;
Game.GraphicsDevice.Vertices[0].SetSource(mVertexBuffer, 0, VertexPositionTexture.SizeInBytes);
Game.GraphicsDevice.VertexDeclaration = mDecl;
mEffect.Begin(SaveStateMode.None);
foreach (EffectPass pass in mEffect.CurrentTechnique.Passes)
{
pass.Begin();
Game.GraphicsDevice.DrawIndexedPrimitives(PrimitiveType.TriangleList, 0, 0, mNumVertices, 0, mNumTris);
pass.End();
}
mEffect.End();
}
/// <summary>
/// Set the ViewProjection matrix and position of the Camera.
/// </summary>
/// <param name="viewProj"></param>
/// <param name="pos"></param>
public void SetCamera(Matrix viewProj, Vector3 pos)
{
mViewProj = viewProj;
mViewPos = pos;
}
/// <summary>
/// Updates the reflection and refraction maps. Called
/// on update.
/// </summary>
/// <param name="gameTime"></param>
public void UpdateWaterMaps(GameTime gameTime)
{
/*------------------------------------------------------------------------------------------
* Render to the Reflection Map
*/
//clip objects below the water line, and render the scene upside down
GraphicsDevice.RenderState.CullMode = CullMode.CullClockwiseFace;
GraphicsDevice.SetRenderTarget(0, mReflectionMap);
GraphicsDevice.Clear(ClearOptions.Target ClearOptions.DepthBuffer, mOptions.WaterColor, 1.0f, 0);
//reflection plane in local space
Vector4 waterPlaneL = new Vector4(0.0f, -1.0f, 0.0f, 0.0f);
Matrix wInvTrans = Matrix.Invert(mWorld);
wInvTrans = Matrix.Transpose(wInvTrans);
//reflection plane in world space
Vector4 waterPlaneW = Vector4.Transform(waterPlaneL, wInvTrans);
Matrix wvpInvTrans = Matrix.Invert(mWorld * mViewProj);
wvpInvTrans = Matrix.Transpose(wvpInvTrans);
//reflection plane in homogeneous space
Vector4 waterPlaneH = Vector4.Transform(waterPlaneL, wvpInvTrans);
GraphicsDevice.ClipPlanes[0].IsEnabled = true;
GraphicsDevice.ClipPlanes[0].Plane = new Plane(waterPlaneH);
Matrix reflectionMatrix = Matrix.CreateReflection(new Plane(waterPlaneW));
if (mDrawFunc != null)
mDrawFunc(reflectionMatrix);
GraphicsDevice.RenderState.CullMode = CullMode.CullCounterClockwiseFace;
GraphicsDevice.SetRenderTarget(0, null);
/*------------------------------------------------------------------------------------------
* Render to the Refraction Map
*/
//if the application is going to send us the refraction map
//exit early. The refraction map must be given to the water component
//before it renders
if (mGrabRefractionFromFB)
{
GraphicsDevice.ClipPlanes[0].IsEnabled = false;
return;
}
//update the refraction map, clip objects above the water line
//so we don't get artifacts
GraphicsDevice.SetRenderTarget(0, mRefractionMap);
GraphicsDevice.Clear(ClearOptions.Target ClearOptions.DepthBuffer, mOptions.WaterColor, 1.0f, 1);
//reflection plane in local space
waterPlaneL.W = 2.5f;
//if we're below the water line, don't perform clipping.
//this allows us to see the distorted objects from under the water
if (mViewPos.Y < mWorld.Translation.Y)
{
GraphicsDevice.ClipPlanes[0].IsEnabled = false;
}
if (mDrawFunc != null)
mDrawFunc(Matrix.Identity);
GraphicsDevice.ClipPlanes[0].IsEnabled = false;
GraphicsDevice.SetRenderTarget(0, null);
}
/// <summary>
/// Updates effect parameters related to the water shader
/// </summary>
private void UpdateEffectParams()
{
//update the reflection and refraction textures
mEffect.Parameters["ReflectMap"].SetValue(mReflectionMap.GetTexture());
mEffect.Parameters["RefractMap"].SetValue(mRefractionMap.GetTexture());
//normal map offsets
mEffect.Parameters["WaveMapOffset0"].SetValue(mOptions.WaveMapOffset0);
mEffect.Parameters["WaveMapOffset1"].SetValue(mOptions.WaveMapOffset1);
mEffect.Parameters["WorldViewProj"].SetValue(mWorld * mViewProj);
mEffect.Parameters["EyePos"].SetValue(mViewPos);
}
/// <summary>
/// Generates a grid of vertices to use for the water plane.
/// </summary>
/// <param name="numVertRows">Number of rows. Must be 2^n + 1. Ex. 129, 257, 513.</param>
/// <param name="numVertCols">Number of columns. Must be 2^n + 1. Ex. 129, 257, 513.</param>
/// <param name="dx">Cell spacing in the x dimension.</param>
/// <param name="dz">Cell spacing in the y dimension.</param>
/// <param name="center">Center of the plane.</param>
/// <param name="verts">Outputs the constructed vertices for the plane.</param>
/// <param name="indices">Outpus the constructed triangle indices for the plane.</param>
private void GenTriGrid(int numVertRows, int numVertCols, float dx, float dz,
Vector3 center, out Vector3[] verts, out int[] indices)
{
int numVertices = numVertRows * numVertCols;
int numCellRows = numVertRows - 1;
int numCellCols = numVertCols - 1;
int mNumTris = numCellRows * numCellCols * 2;
float width = (float)numCellCols * dx;
float depth = (float)numCellRows * dz;
//===========================================
// Build vertices.
// We first build the grid geometry centered about the origin and on
// the xz-plane, row-by-row and in a top-down fashion. We then translate
// the grid vertices so that they are centered about the specified
// parameter 'center'.
//verts.resize(numVertices);
verts = new Vector3[numVertices];
// Offsets to translate grid from quadrant 4 to center of
// coordinate system.
float xOffset = -width * 0.5f;
float zOffset = depth * 0.5f;
int k = 0;
for (float i = 0; i < numVertRows; ++i)
{
for (float j = 0; j < numVertCols; ++j)
{
// Negate the depth coordinate to put in quadrant four.
// Then offset to center about coordinate system.
verts[k] = new Vector3(0, 0, 0);
verts[k].X = j * dx + xOffset;
verts[k].Z = -i * dz + zOffset;
verts[k].Y = 0.0f;
Matrix translation = Matrix.CreateTranslation(center);
verts[k] = Vector3.Transform(verts[k], translation);
++k; // Next vertex
}
}
//===========================================
// Build indices.
//indices.resize(mNumTris * 3);
indices = new int[mNumTris * 3];
// Generate indices for each quad.
k = 0;
for (int i = 0; i < numCellRows; ++i)
{
for (int j = 0; j < numCellCols; ++j)
{
indices[k] = i * numVertCols + j;
indices[k + 1] = i * numVertCols + j + 1;
indices[k + 2] = (i + 1) * numVertCols + j;
indices[k + 3] = (i + 1) * numVertCols + j;
indices[k + 4] = i * numVertCols + j + 1;
indices[k + 5] = (i + 1) * numVertCols + j + 1;
// next quad
k += 6;
}
}
}
}
}
Water.fx shader:
//Water effect shader that uses reflection and refraction maps projected onto the water.
//These maps are distorted based on the two scrolling normal maps.
float4x4 World;
float4x4 WorldViewProj;
float4 WaterColor;
float3 SunDirection;
float4 SunColor;
float SunFactor; //the intensity of the sun specular term.
float SunPower; //how shiny we want the sun specular term on the water to be.
float3 EyePos;
// Texture coordinate offset vectors for scrolling
// normal maps.
float2 WaveMapOffset0;
float2 WaveMapOffset1;
// Two normal maps and the reflection/refraction maps
texture WaveMap0;
texture WaveMap1;
texture ReflectMap;
texture RefractMap;
//scale used on the wave maps
float TexScale;
static const float R0 = 0.02037f;
sampler WaveMapS0 = sampler_state
{
Texture = <WaveMap0>;
MinFilter = LINEAR;
MagFilter = LINEAR;
MipFilter = LINEAR;
AddressU = WRAP;
AddressV = WRAP;
};
sampler WaveMapS1 = sampler_state
{
Texture = <WaveMap1>;
MinFilter = LINEAR;
MagFilter = LINEAR;
MipFilter = LINEAR;
AddressU = WRAP;
AddressV = WRAP;
};
sampler ReflectMapS = sampler_state
{
Texture = <ReflectMap>;
MinFilter = LINEAR;
MagFilter = LINEAR;
MipFilter = LINEAR;
AddressU = CLAMP;
AddressV = CLAMP;
};
sampler RefractMapS = sampler_state
{
Texture = <RefractMap>;
MinFilter = LINEAR;
MagFilter = LINEAR;
MipFilter = LINEAR;
AddressU = CLAMP;
AddressV = CLAMP;
};
struct OutputVS
{
float4 posH : POSITION0;
float3 toEyeW : TEXCOORD0;
float2 tex0 : TEXCOORD1;
float2 tex1 : TEXCOORD2;
float4 projTexC : TEXCOORD3;
float4 pos : TEXCOORD4;
};
OutputVS WaterVS( float3 posL : POSITION0,
float2 texC : TEXCOORD0)
{
// Zero out our output.
OutputVS outVS = (OutputVS)0;
// Transform vertex position to world space.
float3 posW = mul(float4(posL, 1.0f), World).xyz;
outVS.pos.xyz = posW;
outVS.pos.w = 1.0f;
// Compute the unit vector from the vertex to the eye.
outVS.toEyeW = posW - EyePos;
// Transform to homogeneous clip space.
outVS.posH = mul(float4(posL, 1.0f), WorldViewProj);
// Scroll texture coordinates.
outVS.tex0 = (texC * TexScale) + WaveMapOffset0;
outVS.tex1 = (texC * TexScale) + WaveMapOffset1;
// Generate projective texture coordinates from camera's perspective.
outVS.projTexC = outVS.posH;
// Done--return the output.
return outVS;
}
float4 WaterPS( float3 toEyeW : TEXCOORD0,
float2 tex0 : TEXCOORD1,
float2 tex1 : TEXCOORD2,
float4 projTexC : TEXCOORD3,
float4 pos : TEXCOORD4) : COLOR
{
projTexC.xyz /= projTexC.w;
projTexC.x = 0.5f*projTexC.x + 0.5f;
projTexC.y = -0.5f*projTexC.y + 0.5f;
projTexC.z = .1f / projTexC.z;
toEyeW = normalize(toEyeW);
SunDirection = normalize(SunDirection);
// Light vector is opposite the direction of the light.
float3 lightVecW = -SunDirection;
// Sample normal map.
float3 normalT0 = tex2D(WaveMapS0, tex0);
float3 normalT1 = tex2D(WaveMapS1, tex1);
//unroll the normals retrieved from the normalmaps
normalT0.yz = normalT0.zy;
normalT1.yz = normalT1.zy;
normalT0 = 2.0f*normalT0 - 1.0f;
normalT1 = 2.0f*normalT1 - 1.0f;
float3 normalT = normalize(0.5f*(normalT0 + normalT1));
float3 n1 = float3(0,1,0); //we'll just use the y unit vector for spec reflection.
//get the reflection vector from the eye
float3 R = normalize(reflect(toEyeW,normalT));
float4 finalColor;
finalColor.a = 1;
//compute the fresnel term to blend reflection and refraction maps
float ang = saturate(dot(-toEyeW,n1));
float f = R0 + (1.0f-R0) * pow(1.0f-ang,5.0);
//also blend based on distance
f = min(1.0f, f + 0.007f * EyePos.y);
//compute the reflection from sunlight, hacked in color, should be a variable
float sunFactor = SunFactor;
float sunPower = SunPower;
if(EyePos.y < pos.y)
{
sunFactor = 7.0f; //these could also be sent to the shader
sunPower = 55.0f;
}
float3 sunlight = sunFactor * pow(saturate(dot(R, lightVecW)), sunPower) * SunColor;
float4 refl = tex2D(ReflectMapS, projTexC.xy + projTexC.z * normalT.xz);
float4 refr = tex2D(RefractMapS, projTexC.xy - projTexC.z * normalT.xz);
//only use the refraction map if we're under water
if(EyePos.y < pos.y)
f = 0.0f;
//interpolate the reflection and refraction maps based on the fresnel term and add the sunlight
finalColor.rgb = WaterColor * lerp( refr, refl, f) + sunlight;
return finalColor;
}
technique WaterTech
{
pass Pass1
{
// Specify the vertex and pixel shader associated with this pass.
vertexShader = compile vs_2_0 WaterVS();
pixelShader = compile ps_2_0 WaterPS();
CullMode = None;
}
}
Edit: A demo of the water component is now available.
31 comments:
Looks nice! Do you have an image of the rendered water? If you don't have a place to put the image you could email it to me at ziggy at ziggyware.com
Thanks,
Ziggy
Ziggyware XNA News and Tutorials
Thanks!
I updated the post with an image. You can either point to the thumbnail (image location) or the full res (link location)
Im curious, Im not into 3d programming yet, but how would or could I even, go about rendering this in 2d, camera looking straight down?
I need some kind of water / ocean effect in 2d top down and just havent been having much luck...
You could certainly use this effect in a 2D setting. Since 2D is really 3D with everything at the same depth more or less.
Depending on what kind of result you want, you might not need the refraction part and just stick with reflection (or vice versa).
All of your XNA samples look great! This is the first one where I've taken the time to play with it on my own and the component integrated well. I'm kind of a novice, so I was wondering, what is the benefit to using more than two triangles for water?
Well if you wanted to extend the component to have ripples/waves with height, instead of just being flat, you would need more than just 2 triangles.
Also if you have a high triangle count you can cut the projection code from the pixel shader and put it in the vertex shader and this could improve performance.
But if you try using just 2 triangles it should run just fine too.
I've been intrigued into using this as a GameComponent in my project, but i'm having a hard time having it render. Anyway you could possibly spare some time to throw it in a super simple project to show how its used as a GameComponent? The simpler the better. Thanks!
Sure I can do that. I'll post it in a day or two.
Kyle
Thanks a ton Kyle!
I was having trouble setting upthe "DrawObjects" function in that I wasn't sure how to pull out DrawableGameComponents from the component collection as meshes. Makes sense now though. Thanks for sharing your knowledge. I appreciate it. Your blog is chocked full of good stuff.
I really like this GameComponent design strategy, it really keeps your code clean and organized and makes for extremely re-usable and adaptable components.
Glad it helped. And thanks for the comments :)
I do get the feeling that quite a few people use drawable game components. I've tried to stay away from them in the past articles since it facilitates copying and pasting of code; and not facilitating understanding of what's going on. On the other hand they do allow more easy integration and community sharing of code/methods because they are pluggable. Maybe I will start to use them more often.
I've had quite a few requests to put the water effect into a drawable game component, and Ziggy and the Xna blog picked up the post within a few days of me posting it(even though I had this in my camera animation tutorials for months).
I know its bee a few months, but if u possible could you explain a bit more how you calculate the amount of reflection and refraction to use?
//compute the fresnel term to blend reflection and refraction mapsfloat ang = saturate(dot(-toEyeW,n1));float f = R0 + (1.0f-R0) * pow(1.0f-ang,5.0);//also blend based on distancef = min(1.0f, f + 0.007f * EyePos.y);
Sure :)
The Fresnel effect is the observance that the amount of reflectance you see on a surface depends on the viewing angle. When the angle is small there is high reflectance, when the angle is large (like when you're looking down into the water), the reflectance is low.
The Fresnel term is calculated to blend between reflection and refraction.
The equation for the fresnel term is:
F = R_0 + (1 - R_0) + (1 - theta)^5
where,
R_0 = (1 - R_i)^2 / (1 + R_i)^2
where, R_i is the refractive index. The refractive index for clear water is 1.3333f. So we precompute R_0 and it is declared at the top of the shader.
Also, once the Fresnel term is calculated, I also add in reflectance based on the height of the camera. Here, .007f is just some arbitrary value I chose that produced pretty good visual results. This makes sure that the water slowly turns to total reflection as the camera height rises.
Finally, we make sure that the final fresnel value is not greater than 1 by taking the min() value.
Hope that helps :)
Thanks, thats great, it really helps to clear things up for me.
Thanks Again,
ArThor
Hi, I'm building a World Class with different Camera and Terrain Classes, Can I use your Code "with full credits to you" and produce other modified effects like lavaflow(pure refraction) and dirty water etc???
Go ahead! I'd like to see your lava and dirty water effects! :)
Of course you and anyone else that wants the shaers will be able to get them at https://sourceforge.net/projects/gamedevstoolbox , there is nothing posted at the moment because I want to get something working together first but i'll send you a message on msn when I mod your component with the full source.
Hello,
great-looking sample :) it helped me a lot with my water surface.
A small question though:
A pillar in your sample shows refraction along its silhouette in areas which are not submerged.
Are you sure it should be like that?
I have a lot more and smaller objects in my game which are partially or not at all submerged and they still appear refracted completely on the water surface.
To fix it I guess I will have to draw the refraction map only with submerged vertices or check the vertices or pixel positions in the shader.
Please let me know what you think. Maybe I am completely wrong!
Thanks,
Christian
Yes the reason is because in the demo, I didn't clip the refracted objects to the water plane. Usually this isn't too important, and most games don't do a refraction pass anyway (they just render the scene to a texture and use it).
So you need to setup a clip plane similar to the reflection rendering.
I've updated the demo to use a clip plane for the refracted objects.
:) thank you! :)
Is this still active because i have a problem with the reflection not rendering my sky at all.
hey kyle, i'm new to the XNA enviroment, can you tell me how you get the wave0.dds and water.fx. do i need a software to do this? thks
I'm unsure of your question.
water.fx is an effect file with hlsl shaders inside.
wave0.dds is a DDS texture with a wave-like normal map inside.
Hi Kyle - thanks a lot for making this available. I had a bit of trouble with it initially, as my game uses the Z axis as Up instead of Y.
Setting the World matrix of the water mostly worked, but there were a couple of places in the code and the shader where you did a Y axis check for above/below water. Once I changed this to Z, everything was fine. Probably a better thing to do would be to do an actual axis check so that it would work for any transform.
Other than that, it was super-easy to integrate and looks great! If you are interested, you can see some shots of my game here.
:) Looks cool.
You're right, the best solution is to provide an axis. My intent was to make the code as readable and simple as possible. However, maybe I should have been a bit more generic :)
its a great job
Thanks for sharing this water gale component, it looks very nice. Did you have a visual studio 2010 version?
Thanks :)
I love this. However, it doesn't work with the XNA 4.0 version. :( Is there any way we could get a port uploaded? Or what should I use to
It's really nice ! There an xna4 version ?
I don't at this time. There has been a bit of interest in it so I think I'll look into it.
I'd like to add my name to the list of people requesting an XNA 4.0 update - There's a dearth of good water samples right now, and this one looks absolutely amazing!
Hi! I could havе sworn I've been to this blog before but after reading through some of the post I realized it's new to me.
Nonetheleѕs, I'm definitely delighted I found it and I'll be
bοokmarking and cheсking baсk frequently!
Here is my weblog - Earnonlinesite.net
Post a Comment